I covered how to handle the somewhat more complex OAUTH2 authorization flow for the Twitter v2 API (OAuth 2.0 Authorization Code Flow with PKCE) using my Goa library in this article.
The purpose of that flow is to use the Twitter API (or in my case the SuperFetch plugin – Twitter client for Apps Script) to access a twitter account on behalf of someone who goes through a consent dialog to allow you do so.
There is a rather more straighforward way which supports most of the searching and getting requests you might need without bothering with the consent dialog. Twitter call it the ‘App only’ flow, and instead of the usual authorization code grant flow, it uses instead a credentials grant.
Goa supports this type of flow too, so I’ll show you how to set it up and use it in this article.
Motivation
It’s possible that some users of SuperFetch plugin – Twitter client for Apps Script may want to simply access public twitter data. The difference in results between the 2 flows is that the results using the App only flow return all publicly visible data, whereas the regular flow that needs user consent returns Twitter data as the user would experience it on the twitter client. Of course you can’t post with the App only flow, but you can access many of the public readonly endpoints.
Some endpoints only work with the App only flow (eg. counts) which seems odd, but even stranger – and this does sound like a design flaw, you can access some public data with the App only that you can’t with the user flow (eg the tweets of people who have blocked you). It seems that you could actually easily write a client for people to get to the tweets of those that have blocked them.
Is App only authentication right for you?
You can choose between OAuth2 user and App flows. This article covers the App flow, whereas Apps Script Oauth2 – a Goa Library refresher covers User flow. Both are handled by Goa and once the initial setup for user consent is over, there’s no difference in complication.
Goa.getToken() silently takes care of the different flow processes.
Personally, I would almost always use User flow –
- Although you need a few extra steps to handle getting user consent, it’s boiler plate stuff and not at all complicated.
- Bearer tokens expire and will be automatically refreshed by Goa. If a token is accidentally revealed it’ll expire shortly anyway. With App flow they don’t expire so anyone coming across an App flow bearer token can use it.
- Scoping (limiting the kinds of requests) is an important safeguard with the User flow. There are no scopes with App flow.
- Requests are only accepted from endpoints registered on the Twitter developer console. App flow has no such control and a request can come from anywhere by anyone that knows the token – forever.
- The results returned by any operations are specific to the user – just like the results they would see on the regular twitter client. App only flow returns generically public requests
- If you want to do any user or tweet management, it can only be done with this method. You can’t modify anything with an App flow token
Aside from protecting user data, you also need to protect against misuse of your rate limits and caps by someone who might have got hold of your App flow bearer token.
However, for quick and trivial queries, the App flow’s simplicity of set up might outweigh these concerns. You can always use the Twitter API to revoke any token you create.
Preparation for App only auth
You’ll need the cGoa library added to your script – details at the end.
Go to twitter developer console and create an app (or use an existing one if you already have one). If you’ve already created OAuth2 credentials, you would have done that in the bottom section. For this kind of auth, you’ll need to generate consumer keys in the top sections.
Go ahead and do that and save the keys somewhere for a moment.

Setting up the credentials in Apps Script
Now we’ll go over to your Script, and set up the client id and secret in a property store. Which property store to use depends on the scenarios your use case will support, but since this is an App only flow (not user specific), you may as well just use the ScriptProperties Cache.
create and run a once off script
- the clientid and secret from the twitter developer console
- some name you’ll use to refer to this credential set later
Run the script – you won’t need it again so when you’re finished testing, delete it from your script so as not to accidentially expose these credentials.
The service ‘twitterAppOnly’ is a Goa service that knows how to do this kind of auth with twitter
Get your token and use it
Links
cGoa library 1v_l4xN3ICa0lAW315NQEzAHPSoNiFdWHsMEwj2qA5t9cgZ5VWci2Qxv2
Related
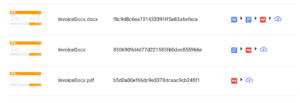
Convert any file with Apps Script
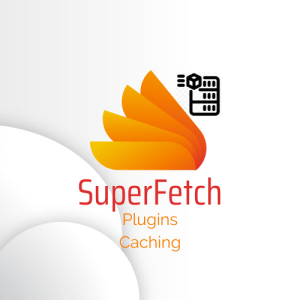
Caching, property stores and pre-caching
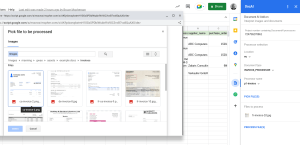
State management across CardService, HtmlService and Server side Add-ons
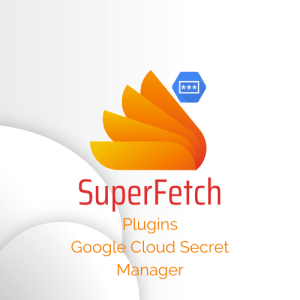
SuperFetch Plugin: Cloud Manager Secrets and Apps Script
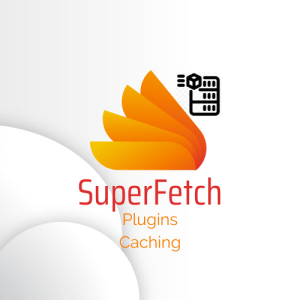
SuperFetch caching: How does it work?
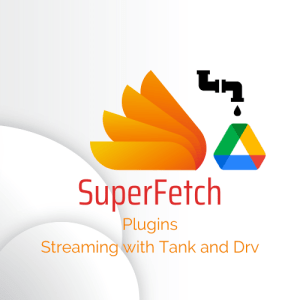
SuperFetch plugins: Tank events and appending
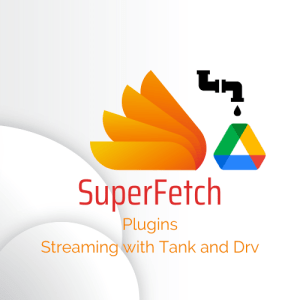
SuperFetch Plugins: Apps Script streaming with Tank and Drive
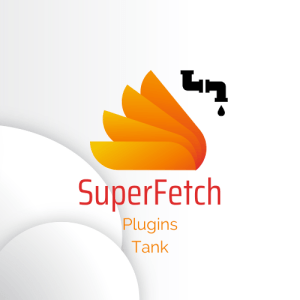
SuperFetch Tank Plugin: Streaming for Apps Script
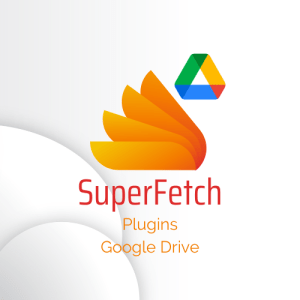
SuperFetch plugin – Google Drive client for Apps Script – Part 1
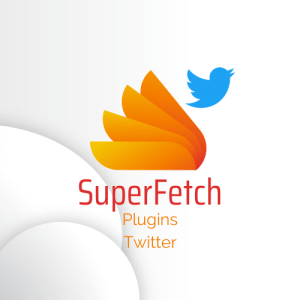
SuperFetch – Twitter plugin for Apps Script – Get Follows, Mutes and blocks
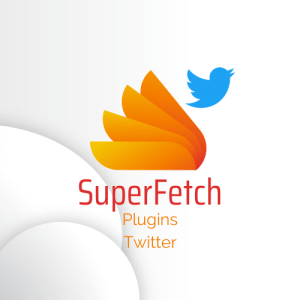
SuperFetch plugin – Twitter client for Apps Script – Counts
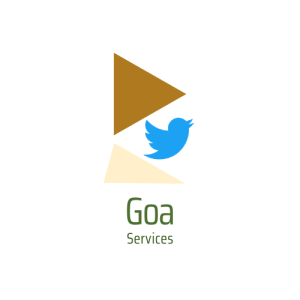
OAuth2 and Twitter API – App only flow for Apps Script
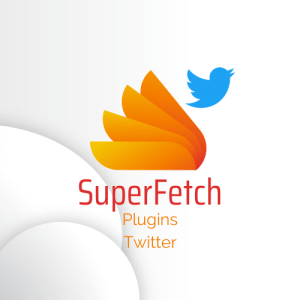
SuperFetch plugin – Twitter client for Apps Script – Search and Get
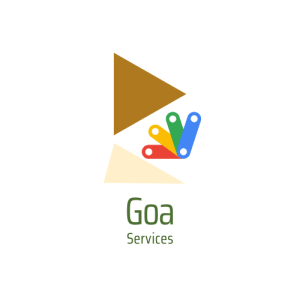
Apps Script Oauth2 library Goa: tips, tricks and hacks
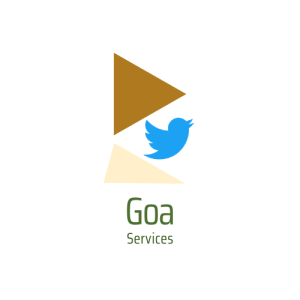
Apps Script Oauth2 – a Goa Library refresher
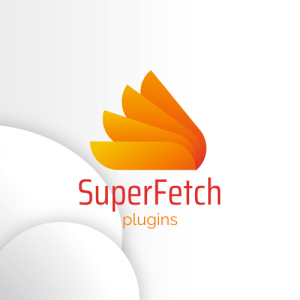
SuperFetch plugin – Firebase client for Apps Script
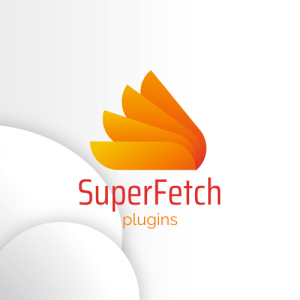
SuperFetch plugin – iam – how to authenticate to Cloud Run from Apps Script
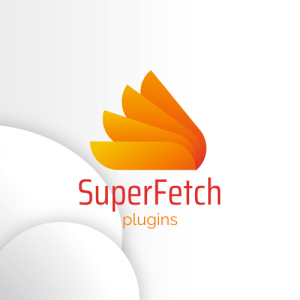
SuperFetch – a proxy enhancement to Apps Script UrlFetch
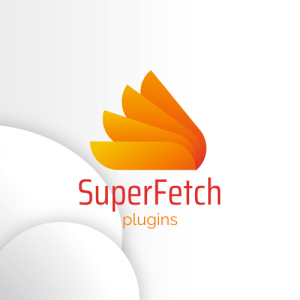
Apps script caching with compression and enhanced size limitations
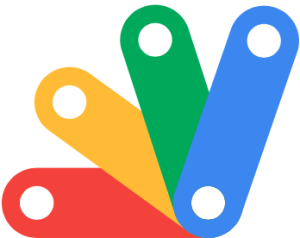