Twt is a SuperFetch plugin to easily access to the Twitter v2 API. SuperFetch is a proxy for UrlFetchApp with additional features – see SuperFetch – a proxy enhancement to Apps Script UrlFetch for how it works and what it does.
This is another in my series on SuperFetch plugins.
Motivation
This is a follow on from SuperFetch plugin – Twitter client for Apps Script – Search and Get but will cover the counts method. This counts the number of occurences of a given query in a period.
Script and Twitter Preparation
You’ll need the bmSuperFetch and cGoa libraries and if you want to run some tests, the bmUnitTest library – details at the end of the article.
Unlike SuperFetch plugin – Twitter client for Apps Script – Search and Get which can use either Twitter Oauth2 User flow or the less secure OAuth2 and Twitter API – App only flow for Apps Script (giving subtly different results), the counts method only accepts queries with the App flow. This is because it accesses purely public data without any specific user filtering.
Instantiation
There’s a few things you need to initialize before you can start running your script
Goa
First you’ll need a Goa to provide a token service – see OAuth2 and Twitter API – App only flow for Apps Script for how to set up. You can have multiple Goas in the same app, so you you can have both User and App only flow enabled.
SuperFetch
You need to include the plugins you need, and an instaniated SuperFetch instance. If you don’t need caching, then just omit the cacheService property (but I highly recommend caching with this API). If you are using both types of OAuth2 tokenService make a superFetch instance for each.
Twt instance
This will be the handle for accessing Twitter.
There are various other standard SuperFetch Plugin parameters that I’ll deal with later, but the ones of most interest are:
superFetch (required)
The instance you created earlier
noCache property (optional)
In this instance, I’m turning caching off for now.
showUrl property (optional)
Normally this would be false, but if you want to see you how your calls to the twt client are translated to native Rest calls, you can add this and set it to true.
Calling structure
The twt plugin uses closures heavily. A closure is a function which carries the references to its state (the lexical environment) with it. This encapsulation is very handy for creating reusable short cuts to standard queries, as we’ll cover later.
Counting
Here’s how you’d get the recent count of tweets that match a particular query . The query is constructed using the same syntax as you’d use in the normal web client. The full details are here.
Responses
All responses have exactly the same format. You’ll get back a standard SuperFetch Response, which looks like this
Twitter API data
The response from the Twitter API will be in the data property of the SuperFetch response.
Actually the twitter response will have been massaged a bit so that every API call has exactly the same format. There is 1 property of interest
- items – an array of standard data from the Twitter API
Data items
The items are an array of basic data matching the search criteria
Closures
You can specify the search query in a number of ways, including creating a query closure.
Additional parameters
You can enhance the results by adding parameters – these are documented in the API reference detail.
The most interesting one is granuarity – (minute, hour or day) – hour is the default
Using .throw
If SuperFetch encounters an error it’ll be in the error property of the response
- you can handle this yourself by checking the response error property.
- The plugin can automatically throw an error on your behalf if you can add the .throw() method to any request. All SuperFetch plugins have the same error handling approach. Think of it as a built in try/catch.
Caching
Caching is built into SuperFetch (for details see SuperFetch – a proxy enhancement to Apps Script UrlFetch) so you get caching out of the box. For more details on caching with this api see SuperFetch plugin – Twitter client for Apps Script – Search and Get
Recent and All
- recent – tweets from the last 7 days
- all – all the twitter archive
The plugin supports both, but – from the API docs regarding the ‘all’ endpoint.
“This endpoint is only available to those users who have been approved for Academic Research access.
The full-archive Tweet counts endpoint returns the count of Tweets that match your query from the complete history of public Tweets; since the first Tweet was created March 26, 2006.”
Recent
This is the default, and you never need to specify it. For completeness it looks like this
All
Only Academic researchers have access to this, and most of us will get this error – which strangely enough is not an accurate description of the error anyway.
Unit testing
I’ll use Simple but powerful Apps Script Unit Test library to demonstrate calls and responses. It should be straightforward to see how this works and the responsese to expect from calls. These tests demonstrate in detail each of the topics mentioned in this article, and a few others, and could serve as a useful crib sheet for the plugin
Links
bmSuperFetch: 1B2scq2fYEcfoGyt9aXxUdoUPuLy-qbUC2_8lboUEdnNlzpGGWldoVYg2
bmUnitTester: 1zOlHMOpO89vqLPe5XpC-wzA9r5yaBkWt_qFjKqFNsIZtNJ-iUjBYDt-x
cGoa library 1v_l4xN3ICa0lAW315NQEzAHPSoNiFdWHsMEwj2qA5t9cgZ5VWci2Qxv2
Related
Twitter API docs https://developer.twitter.com/en/docs/twitter-api
Twitter Developer profile https://developer.twitter.com/en/portal
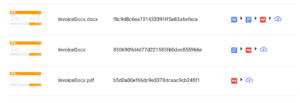
Convert any file with Apps Script
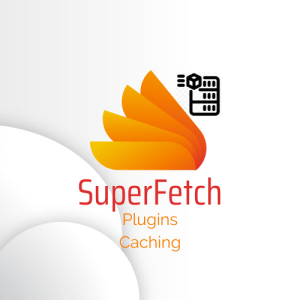
Caching, property stores and pre-caching
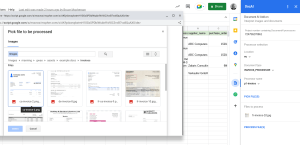
State management across CardService, HtmlService and Server side Add-ons
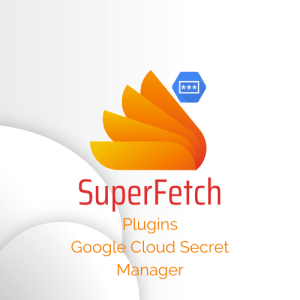
SuperFetch Plugin: Cloud Manager Secrets and Apps Script
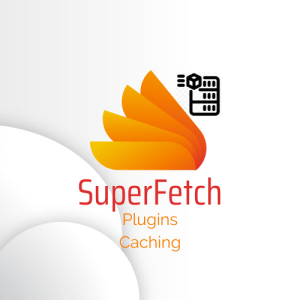
SuperFetch caching: How does it work?
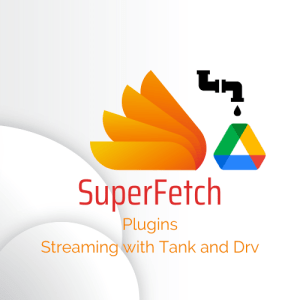
SuperFetch plugins: Tank events and appending
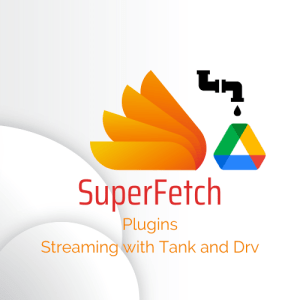
SuperFetch Plugins: Apps Script streaming with Tank and Drive
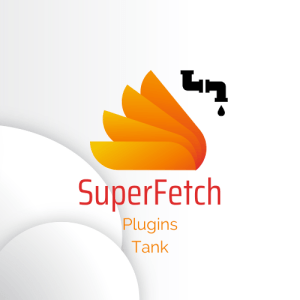
SuperFetch Tank Plugin: Streaming for Apps Script
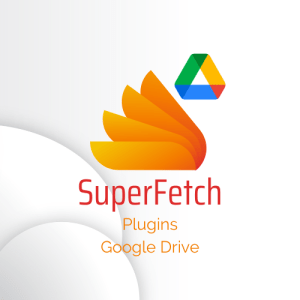
SuperFetch plugin – Google Drive client for Apps Script – Part 1
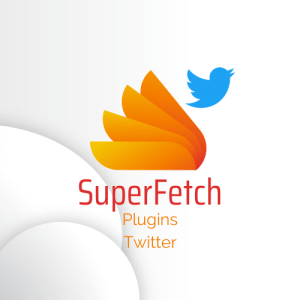
SuperFetch – Twitter plugin for Apps Script – Get Follows, Mutes and blocks
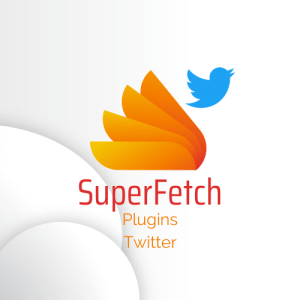
SuperFetch plugin – Twitter client for Apps Script – Counts
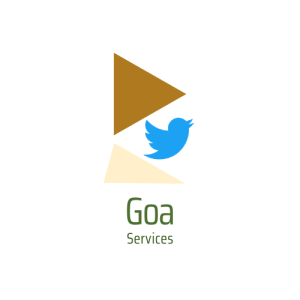
OAuth2 and Twitter API – App only flow for Apps Script
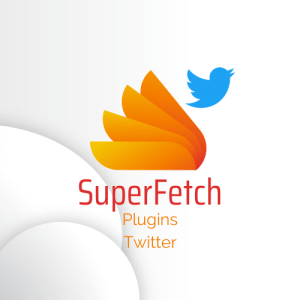
SuperFetch plugin – Twitter client for Apps Script – Search and Get
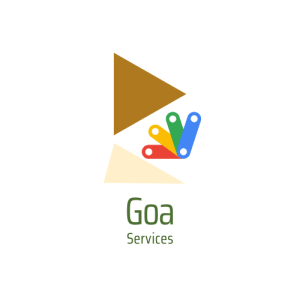
Apps Script Oauth2 library Goa: tips, tricks and hacks
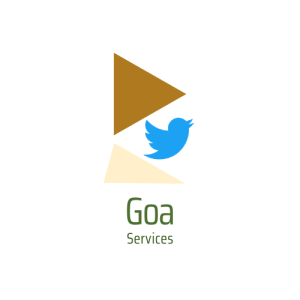
Apps Script Oauth2 – a Goa Library refresher
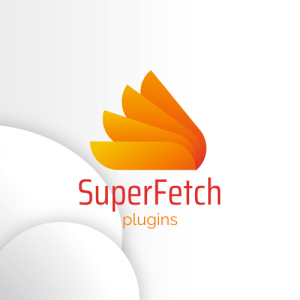
SuperFetch plugin – Firebase client for Apps Script
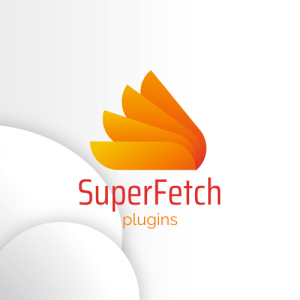
SuperFetch plugin – iam – how to authenticate to Cloud Run from Apps Script
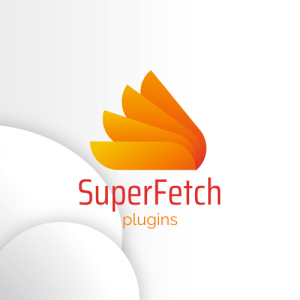
SuperFetch – a proxy enhancement to Apps Script UrlFetch
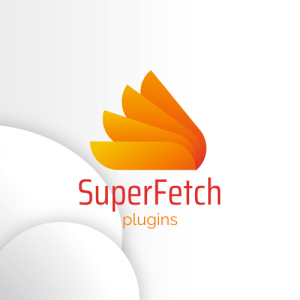
Apps script caching with compression and enhanced size limitations
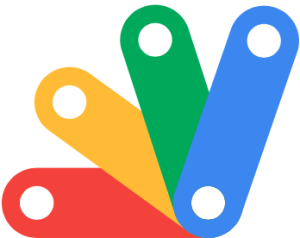