Twt is a SuperFetch plugin to easily access to the Twitter v2 API. SuperFetch is a proxy for UrlFetchApp with additional features – see SuperFetch – a proxy enhancement to Apps Script UrlFetch for how it works and what it does.
This is another in my series on SuperFetch plugins. It also includes a script to analyze your twitter circle to a spreadsheet.
Motivation
This is a follow on from SuperFetch plugin – Twitter client for Apps Script – Search and Get but will cover the followers, following, muting and blocking methods. This returns user data for each of those endpoints
Script and Twitter Preparation
You’ll need the bmSuperFetch and cGoa libraries and if you want to run some tests, the bmUnitTest library – details at the end of the article.
Like SuperFetch plugin – Twitter client for Apps Script – Search and Get you can use either Twitter Oauth2 User flow or the less secure OAuth2 and Twitter API – App only flow for Apps Script
I recommend using the user Flow where you can.
Instantiation
There’s a few things you need to initialize before you can start running your script
Goa
First you’ll need a Goa to provide a token service – see Twitter Oauth2 User flow for how to set up. All the code you need is below. Just patch in your credentials, run the onceoff, deploy as a webapp and use test deployment to get the consent process kicked off. If you’ve done all this before for previous endpoints, note that there’s a couple of extra scopes you’ll need in the goaPackage for these methods.
SuperFetch
Include the plugins you need, and an instaniated SuperFetch instance. If you don’t need caching, then just omit the cacheService property (but I highly recommend caching with this API).
Twt instance
This will be the handle for accessing Twitter.
There are various other standard SuperFetch Plugin parameters that I’ll deal with later, but the ones of most interest are:
superFetch (required)
The instance you created earlier
noCache property (optional)
In this instance, I’m turning caching off for now.
showUrl property (optional)
Normally this would be false, but if you want to see you how your calls to the twt client are translated to native Rest calls, you can add this and set it to true.
Calling structure
The twt plugin uses closures heavily. A closure is a function which carries the references to its state (the lexical environment) with it. This encapsulation is very handy for creating reusable short cuts to standard queries, as we’ll cover later.
Get my id
We’ll be using my id for theses test, so first here’s how to get your own record
Get followers
Here’s how to get a list of users that follow you.
Get following
Here’s how to get a list of users that you follow.
Get blocking
Here’s how to get a list of users that you have blocked
Get muting
Here’s how to get a list of users that you have muted
Example
Let’s say we want to find out which users I follow who are also following me back, those that are following me but that I don’t follow back, and those that I follow but are not following me back, plus the number I’ve blocked and muted.
Rate limit
At this point, depending on how many followers etc you have, you’re likely to hit a rate limit problem.
Using Superfetch’s built in caching is a great way to minimize the number of API requests you make, but when cache has expired (you can set the expiry time as a SuperFetch parameter – by default it’s 1 hour) or it’s the first time you make a particular query, it’ll count against your rate limit. The full story on Twitter rate limits is here
There are a number of ways of dealing with rate limits, some of which I’ve already covered in other SuperFetch articles, but the twitter one gives some clues to help so we don’t have to rely on exponential backoff or throttling calls as described in SuperFetch – a proxy enhancement to Apps Script UrlFetch
In the case of these user endpoints, twitter only allows 15 requests in a 15 minute period. Unlike tweet searching (which only allows only 100 reponses per page), these allow 1000 responses per page, so the Twt plugin will automatically adjust the request pagesize to the maximum it can get away with for each endpoint.
The plugin also returns a special rateLimit property as a response to each query which gives info about how much you have left – but that’ll be the subject of another SuperFetch/Twt article.
Using public metrics
In the previous example, we’ve retrieved the data for each of the users following etc. to get the counts. That’s because we need to analyze the intersection between them. If you just want the raw counts, there’s a much easier way using the user’s public metrics
Here’s how we can request some additional fields about your own profile. Of course this only gives a couple of summaries, but perhaps it’s enough
Writing the lists to a sheet
Since we’re in Apps Script here, we as may well get some more info about our followers and write it all to a sheet. I’m going to use the bmPreFiddler library for this, as it makes sheet manipulation a bit easier. ID details at end of post.
That gives us a sheet of everyone in your twitter universe along with their stats and relationship to you. It’ll make a a good crib sheet for a twitter clearout.

Unit testing
I’ll use Simple but powerful Apps Script Unit Test library to demonstrate calls and responses. It should be straightforward to see how this works and the responsese to expect from calls. These tests demonstrate in detail each of the topics mentioned in this article, and a few others, and could serve as a useful crib sheet for the plugin
Links
bmSuperFetch: 1B2scq2fYEcfoGyt9aXxUdoUPuLy-qbUC2_8lboUEdnNlzpGGWldoVYg2
bmUnitTester: 1zOlHMOpO89vqLPe5XpC-wzA9r5yaBkWt_qFjKqFNsIZtNJ-iUjBYDt-x
cGoa library 1v_l4xN3ICa0lAW315NQEzAHPSoNiFdWHsMEwj2qA5t9cgZ5VWci2Qxv2
bmPreFiddler (13JUFGY18RHfjjuKmIRRfvmGlCYrEkEtN6uUm-iLUcxOUFRJD-WBX-tkR)
Related
Twitter API docs https://developer.twitter.com/en/docs/twitter-api
Twitter Developer profile https://developer.twitter.com/en/portal
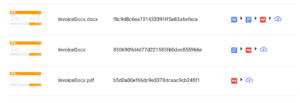
Convert any file with Apps Script
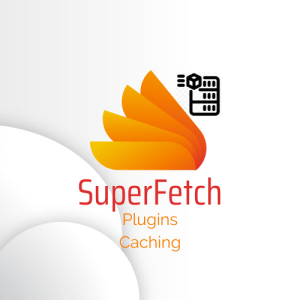
Caching, property stores and pre-caching
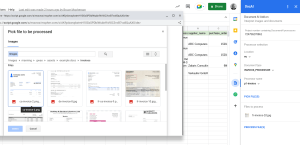
State management across CardService, HtmlService and Server side Add-ons
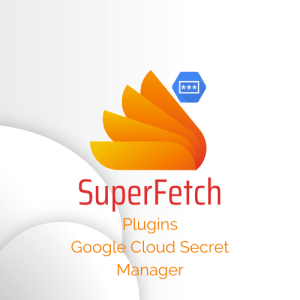
SuperFetch Plugin: Cloud Manager Secrets and Apps Script
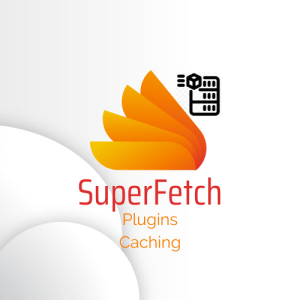
SuperFetch caching: How does it work?
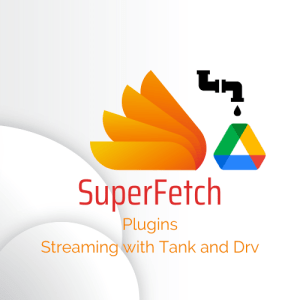
SuperFetch plugins: Tank events and appending
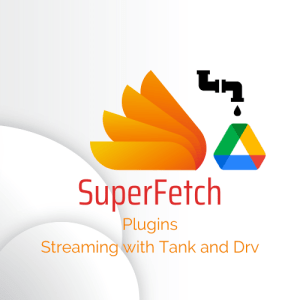
SuperFetch Plugins: Apps Script streaming with Tank and Drive
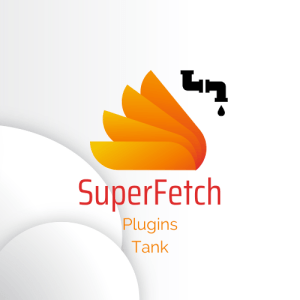
SuperFetch Tank Plugin: Streaming for Apps Script
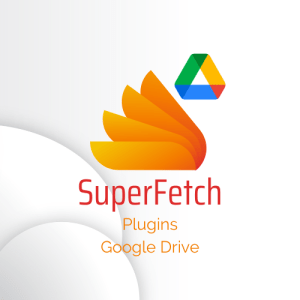
SuperFetch plugin – Google Drive client for Apps Script – Part 1
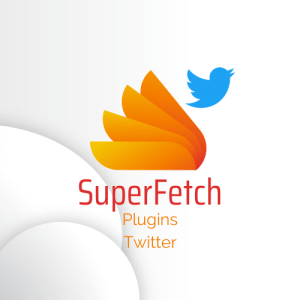
SuperFetch – Twitter plugin for Apps Script – Get Follows, Mutes and blocks
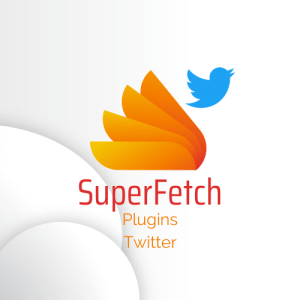
SuperFetch plugin – Twitter client for Apps Script – Counts
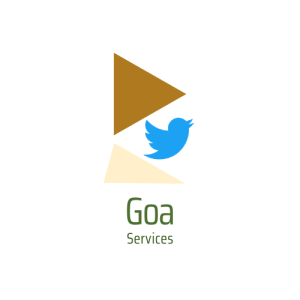
OAuth2 and Twitter API – App only flow for Apps Script
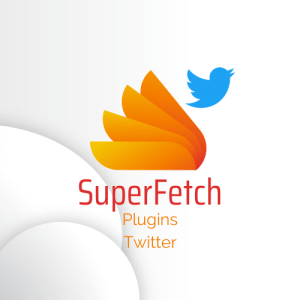
SuperFetch plugin – Twitter client for Apps Script – Search and Get
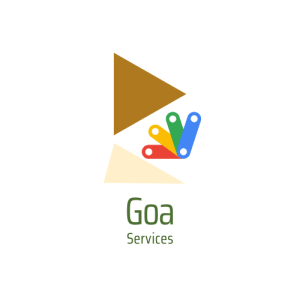
Apps Script Oauth2 library Goa: tips, tricks and hacks
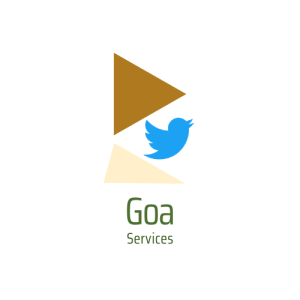
Apps Script Oauth2 – a Goa Library refresher
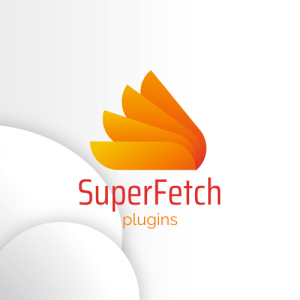
SuperFetch plugin – Firebase client for Apps Script
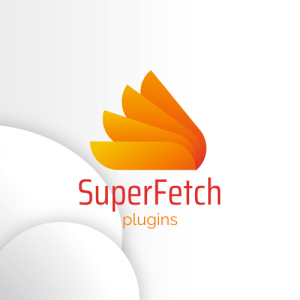
SuperFetch plugin – iam – how to authenticate to Cloud Run from Apps Script
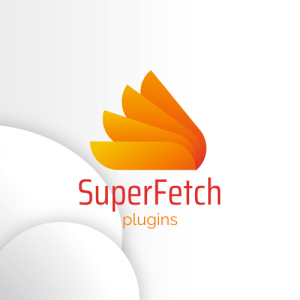
SuperFetch – a proxy enhancement to Apps Script UrlFetch
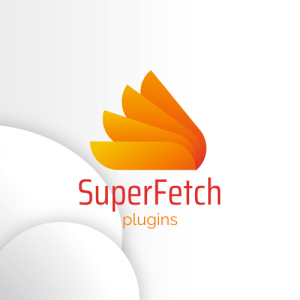
Apps script caching with compression and enhanced size limitations
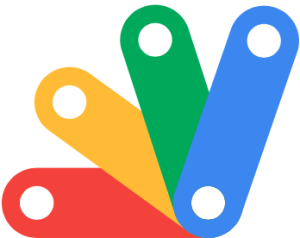