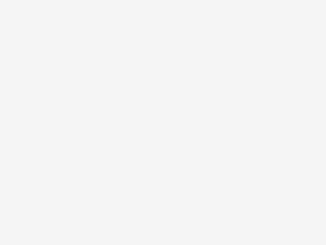
Reporting file, function and line number in Apps Script
If you are using the Logger extensively, it’s sometimes hard to track where the log message is coming from. It would be useful to display line number, file name and function that provoked the log. […]