So it turns out that Slideshare has an API that you can do various things with including getting stats, so I though I may as well use that. I was expecting it to use OAuth2 but it doesn’t. It does have a client secret and an API key it uses to measure your usage (pretty low daily quota actually), but the most useful stuff (for me anyway) doesn’t need authentication – (which is done through a username.password approach)
The data also comes back in XML format, so I’ve created an Apps Script library to convert that back into JSON and to handle the API interaction. For now I’ve only implemented a couple of methods
– getSlideshowsByUserName
– getSlideShow
I may add to that later if anybody is interested.
Set up
You need to apply for an apikey and client secret at the slideshare API page, but it’s painless – you get one by email right away. Once you have those, I recommend you store them in a properties store somewhere.
You’ll need the cSlideShare library (or you can get in from github)
17BGwrmAH670Wo4-CNibKixJLsFA3Zk4D4XucgLDTcbbuuov0rOgSGMCM
Then you can do this .. or something else if you haven’t stored your credentials in a properties store as I have,
// get all my slideshare stuff // script properties have my creds var sshareCreds = JSON.parse(PropertiesService.getScriptProperties().getProperty("slideshareCredentials")); var sshare = new cSlideShare.SlideShare() .setSecret(sshareCreds.secret) .setApiKey(sshareCreds.apiKey) .setUserName(sshareCreds.userName)
Now you can get all your (or someone elses) slideshare data like this (the true says I want maximum detail)
// get all of them .. the first arg can be any slideshare username var all = sshare.getSlideshowsByUserName ("",true).data;
Or you can get the data for one slideshow like this
// ...or get just one by its ID var one = sshare.getSlideshow ("55548998",true).data;
and that’s pretty much it. Here’s how to select a few fields and write the data to a sheet
// dump to a sheet var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName('slides'); sheet.clearContents(); // there are loads of fields, but i'll just take these for demo. var fields = ["ID","Title","URL","Created", "NumViews", "NumSlides"]; var values = [fields].concat(all.Slideshow.map (function (d) { return fields.map (function (f) { return d[f]; }); })); // write that out sheet.getRange (1,1,values.length,fields.length).setValues(values); }
Which gives you something like this
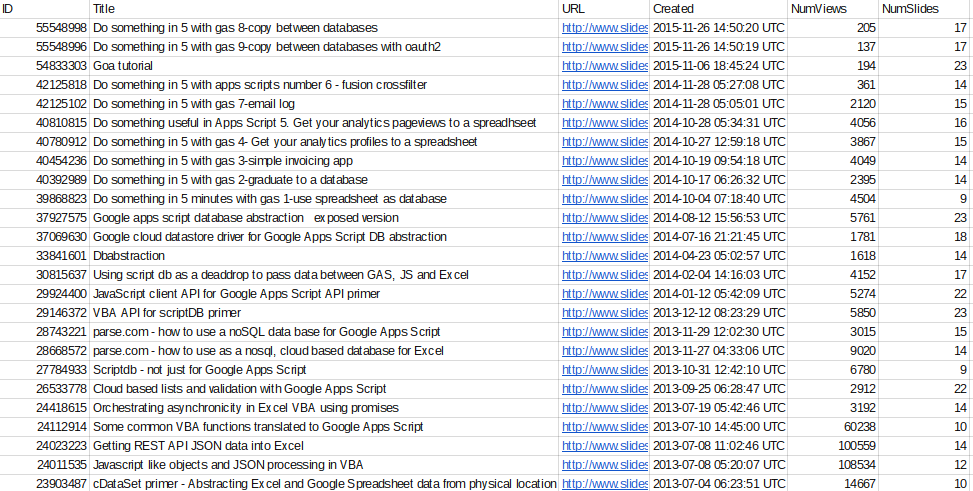